Overview
This portfolio serves to document my contributions to a team-based software engineering project for the module CS2113/T, Software Engineering and Object-Oriented Programming. My team comprised 4 members, including myself, and we were tasked with morphing or enhancing the Addressbook Level 4 project (AB4) by SE-EDU within 8 weeks. We decided to morph AB4 into a task management app named Student Buddy.
Student Buddy is a desktop application tailored to the needs of students at the National University of Singapore. Specifically, it is meant for students who are comfortable with using a Command Line Interface (CLI). The use of a CLI for user input allows users to input commands more efficiently than when using a conventional user interface, thereby allowing them to better manage their tasks and their time. The app does, however, retain a Graphical User Interface (GUI) for easy viewing of information.
The project is written in Java, with JavaFX used to create the GUI.
Summary of contributions
The following list is a summary of my contributions to the project Student Buddy:
-
Major enhancement: added a calendar panel to the GUI for easy viewing of tasks and their deadlines.
-
What it does: allows the user to view their tasks in a format that is familiar and easy to read. In addition, tasks are ordered and colour-coded by priority (high to low), allowing users to easily see which tasks are more urgent.
-
Justification: This feature improves the product significantly because a user is able to quickly and easily see what tasks are due and when, facilitating better time management.
-
Highlights: This enhancement piggybacks off of existing infrastructure, so future changes to underlying systems may cause the enhancement to break. It required an in-depth study of new concepts, as GUI modification was completely new to me before this project. I also wrote tests to ensure that the enhancement was working correctly.
-
-
Minor enhancement: added a
month
command to the app-
What it does: allows the user to change the month displayed on the calendar
-
-
Code contributed: RepoSense
-
Other contributions:
The following is a list of my other contributions to the project, besides the features I implemented. Relevant pull requests are listed beside each entry, if applicable.-
Project management:
-
Set up milestones in the team repo for tracking our progress on the project
-
Set up tags for the team repo’s issue tracker
-
Deployed release v1.3.1
-
-
Enhancements to other features:
-
Assisted in adding a due date display to the task card list in the GUI (#94)
-
Assisted in formatting and presentation of notes function in the GUI (#119)
-
Added functionality to select feature, causing selected tasks to be cleared upon modification of the task list (#166)
-
Assisted in fixing bugs and writing tests for other features (#176)
-
Adjusted the GUI as features were added and modified, whether by myself or teammates (#94, #119, #166)
-
-
Documentation:
-
Community:
-
Tools:
-
Integrated Coveralls to the team repo
-
-
Contributions to the User Guide
While the AB4 project came with a User Guide, our team had to modify it extensively to match our product. For my part, I wrote about the features of my calendar panel, and how users could use it. I also wrote about my month changing feature. Below is a sample of my work. |
Viewing tasks on a calendar
Tasks displayed in the task list are automatically displayed on a calendar of the current month.
In addition, the calendar comes with the following features:
-
Tasks are automatically colour-coded, with high, medium and low priority tasks appearing red, orange and blue, respectively.
-
Tasks are automatically listed in order of priority, from high to low.
-
If the task list is modified with
add
,delete
oredit
, or it is filtered usingfind
, the calendar will update accordingly. -
Selecting a task will highlight it on the calendar for easy viewing.
-
Calendar cells are scrollable.
A showcase of the calendar’s features is displayed below:
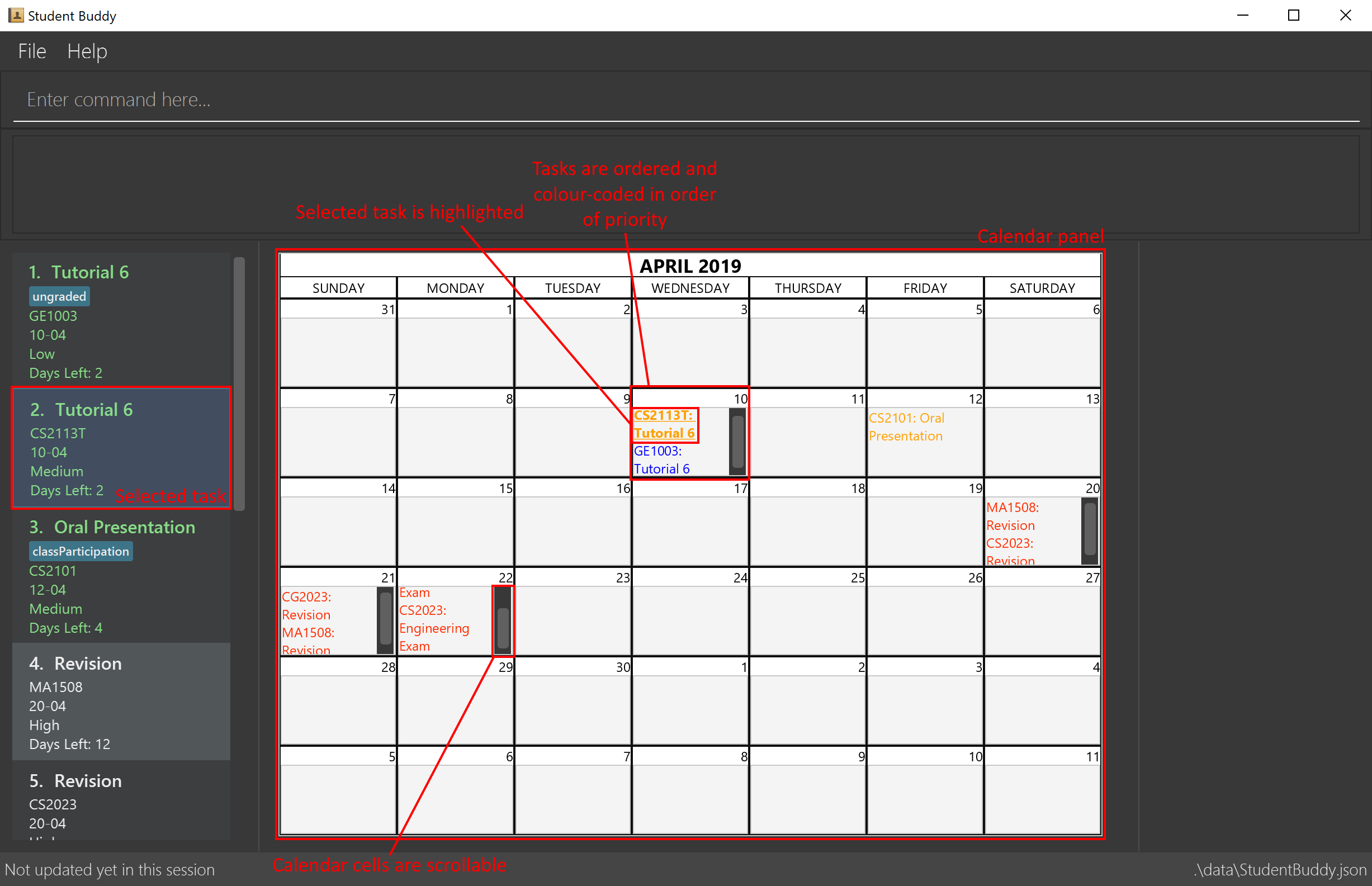
Contributions to the Developer Guide
The AB4 project also came with a Developer Guide, which we also modified to match our project. For my part, I wrote about the implementation of my calendar panel and the month changing feature. I also contributed towards cleaning up some grammar mistakes in the guide, as well as adjusting the tone and formatting to be more consistent. Below is a sample of my contributions. |
Calendar feature
Current Implementation
The Calendar extends the Student Buddy
GUI with an easy to read interface for tracking task deadlines. It is composed of three classes, CalendarPanel
, CalendarCell
and CalendarCellTask
. Furthermore, it uses the JavaFX files CalendarPanel.fxml
and CalendarCell.fxml
to format and structure the display.
CalendarPanel
is the base class, which builds and fills the calendar grid.
CalendarCell
represents an individual cell of the grid in CalendarPanel
.
CalendarCellTask
represents an individual task inside each CalendarCell
.
CalendarPanel.fxml
is a ScrollPane
containing a GridPane
. The GridPane
acts as the calendar grid.
CalendarCell.fxml
is a VBox
containing a Text
, and a ScrollPane
containing another VBox
. The Text
is the date of a calendar cell, and the second VBox
contains the list of tasks in a cell.
The following class diagram illustrates the relationships between CalendarPanel
, CalendarCell
and CalendarCellTask
:
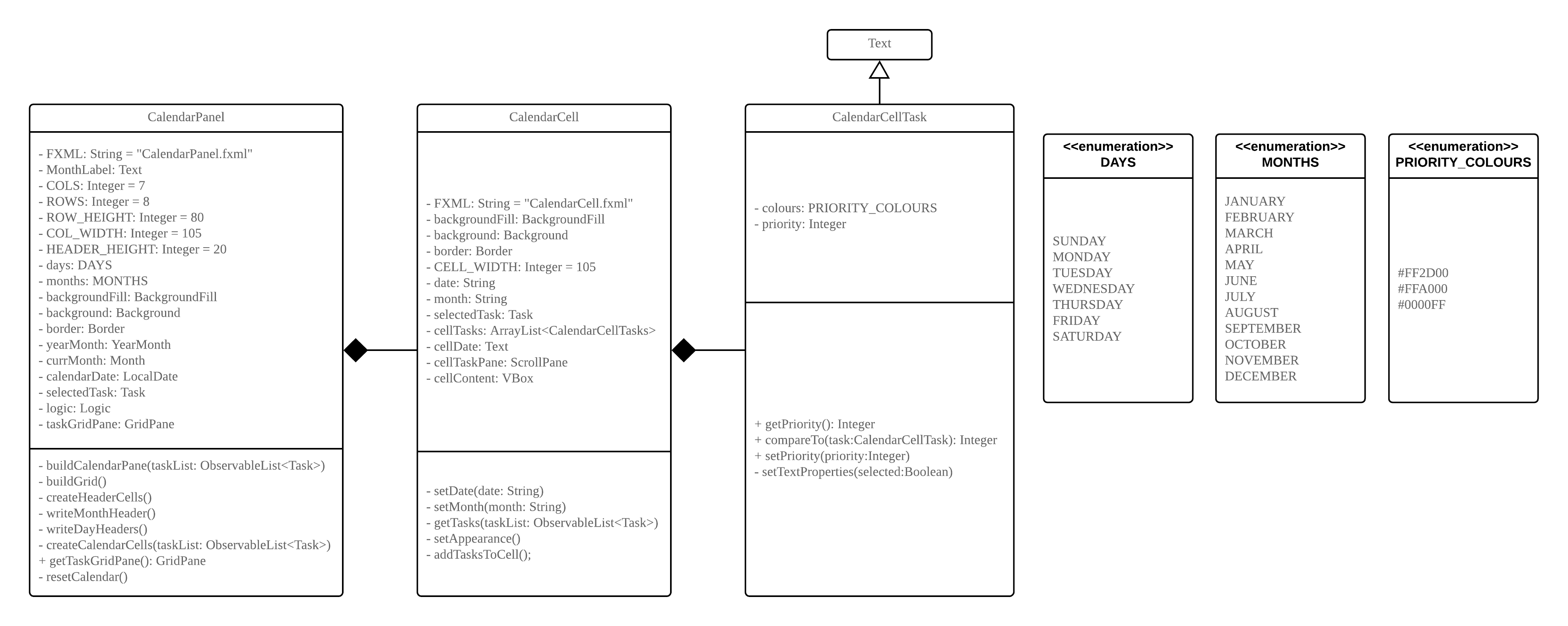
The following steps show how the Calendar is built on startup:
Step 1: The constructor of |
Step 2: |
Step 3: |
Step 4: |
Step 5: |
Step 6: |
Step 7:
|
Step 8: Done. |
The following sequence diagram illustrates the process outlined above:
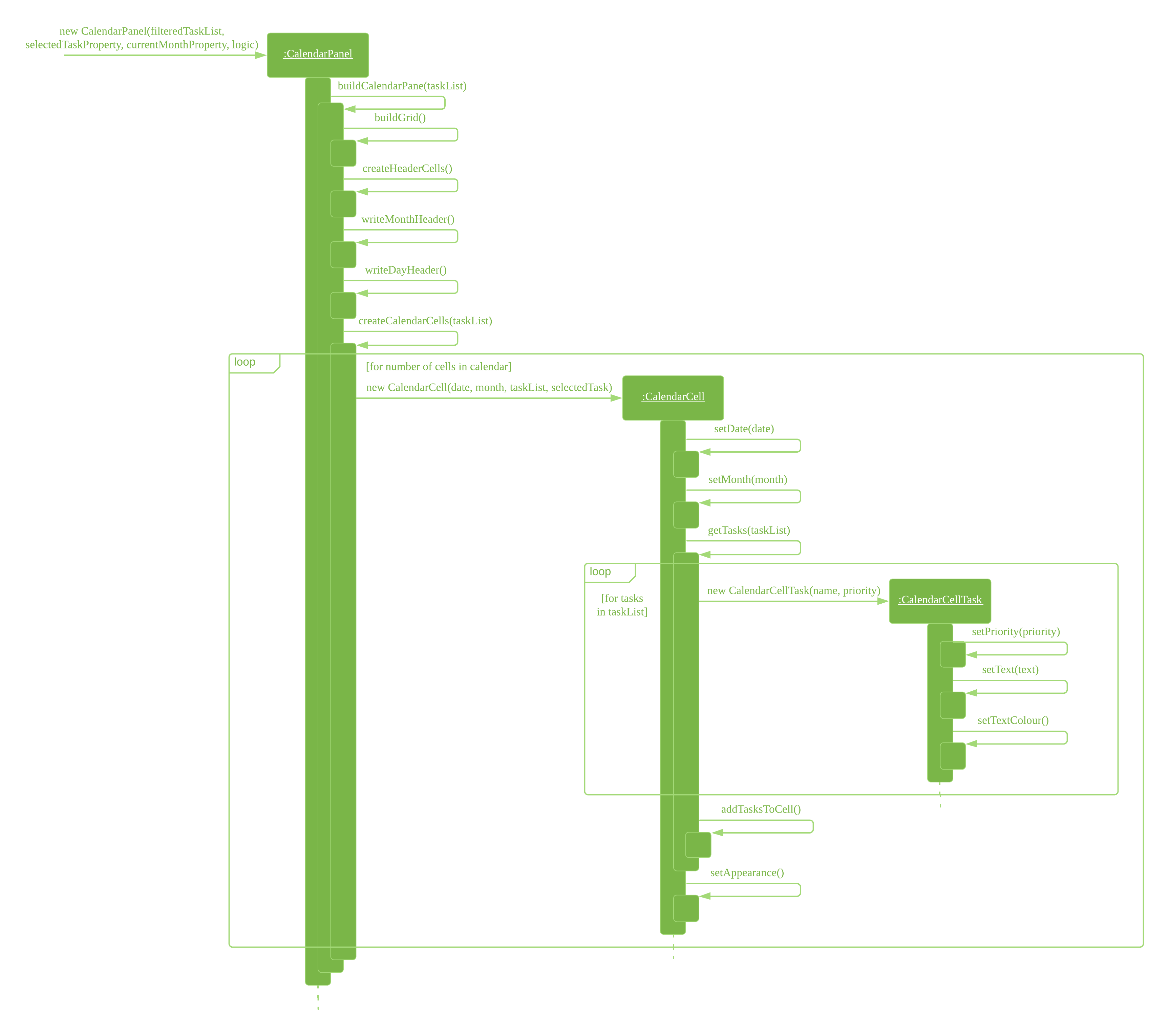
Whenever the task list is updated, the function createCalendarCells(taskList)
is called, which replaces the CalendarCell
and CalendarCellTask
instances in the CalendarPanel
.
If the selected task is changed, or the month to be displayed changes (see [Month view change feature]), the function resetCalendar()
is called, which clears the calendar grid and resets the row and column constraints. Then buildCalendarPane(taskList)
is called to rebuild the calendar.
Design Considerations
Aspect: How the Calendar is built
-
Alternative 1 (current choice): Separate the calendar panel, calendar cells and tasks into their own classes.
-
Pros: Reduces complexity of CalendarPanel class, making it easier to understand how the calendar is built.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Separate every component of the Calendar into their own classes (e.g. into CalendarPane, CalendarGrid, HeaderCell, ContentCell, etc).
-
Pros: Follows the principles of Single Responsibility Principle and Separation of Concerns strictly.
-
Cons: Even more memory usage, may make the code difficult to read and understand for future maintainers if they are unused to code spanning several files.
-
-
Alternative 3: Combine all Calendar related code into a single class.
-
Pros: No need to navigate between different classes.
-
Cons: The class will be very long and complex, making the code difficult for future maintainers to read, understand and change. Violates the principles of Single Responsibility Principle and Separation of Concerns.
-
-
Rationale for choice:
-
It is the middle ground between alternatives 2 and 3, and thus strikes a balance between readability, maintainability and following Object-Oriented Programming principles. While it does not strictly follow the principles of OOP, it is easy to read the code and understand the processes involved, and is maintainable. This is important, as it is likely that future maintainters will be new Computer Science student undergraduates.
-
Aspect: How the calendar is updated in real time
-
Alternative 1 (current choice): Replace the previous
CalendarCell
andCalendarCellTask
instances with with new instances when the task list changes.-
Pros: Easy to read and to understand, simpler and easier to implement.
-
Cons: Potential performance issues. If the list of tasks is very large, rebuilding the Calendar at every step may result in degraded performance manifested as a loss of responsiveness to user commands.
-
-
Alternative 2: Have
CalendarCell
andCalendarCellTask
instances automatically update as the task list changes.-
Pros: No need to rebuild the entire calendar when the task list changes, instead only updating the cells and tasks that are affected.
-
Cons: Adds another layer of abstraction, which can cause difficulty in understanding how the Calendar works.
-
-
Rationale for choice:
-
The choice of alternative 1 was made due to time constraints and lack of proper understanding of how to implement alternative 2. Ideally, alternative 2 will be implemented by future maintainers.
-